Class Diagrams
A Class diagram gives an overview of a system by showing its classes and the relationships among them. Class diagrams are static -- they display what interacts but not what happens when they do interact.
The class diagram below models a customer order from a retail catalog. The central class is the Order. Associated with it are the Customer making the purchase and the Payment. A Payment is one of three kinds: Cash, Check, or Credit. The order contains OrderDetails (line items), each with its associated Item.
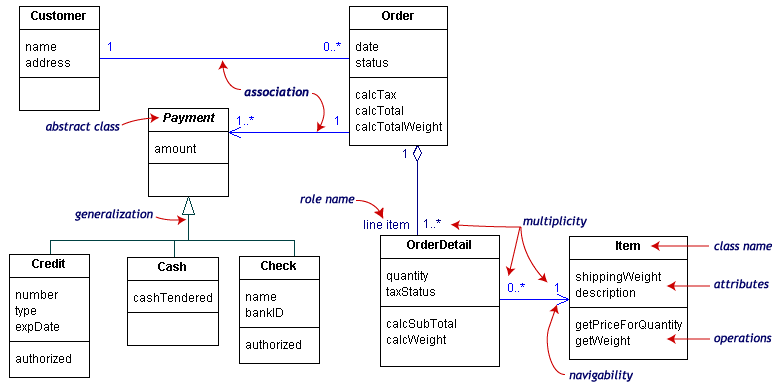
UML class notation is a rectangle divided into three parts: class name, attributes, and operations. Names of abstract classes, such as Payment, are in italics. Relationships between classes are the connecting links.
In UML a feature characterizes the instances of a classifier or the classifier itself.
- Structural features are properties (attributes, association ends, parts, roles) and behavioral features are operations of a class.
- Part (see examples in Composite Structure Diagrams below)
An element representing a set of instances by composition that are owned by a containing classifier instance or role of a classifier (compare to role below). All such instances are destroyed when the containing classifier is destroyed. Parts may be joined by attached connectors and specify configurations of linked instances to be created within an instance of the containing classifier. - Role
The named set of features defined over a collection of entities participating in a particular context, usually specified by interfaces that will prescribe properties that the participating instances must exhibit.
Collaboration: The named set of collaborating elements (roles) each performing a specialized function, which collectively accomplish some desired functionality.
Part: a subset of a particular class which exhibits a subset of features possessed by the class
Associations: A synonym for association end often referring to a subset of classifier instances that are participating in the association.
Attributes
- The form of an attribute is: visibility / name : prop-type [multiplicity] = default {prop-string, ...}
- Only name is necessary
- visibility ::= { -, +, #, ~}private, public, protected, package
- multiplicity ::= [lower..upper]The multiplicity of an attribute specifies how many instances of the attribute's type are created when the owning class is instantiated.
- / denoted the attribute is derived. A derived attribute is simply one that can be computed from other attributes.
- An example of this is: - name: String [1] = "Untitled" {readonly}
Attributes by Relationship
- Inlined attributes can be represented using the relationship notation.
- It can provide greater detail for complex attribute types.
- The relationship notation also conveys exactly how the attribute is contained within a class.
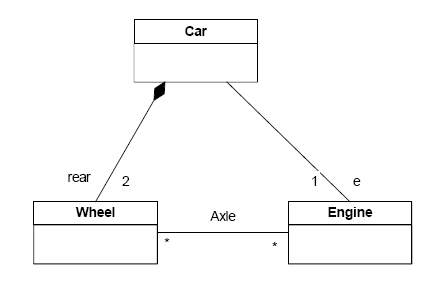
Operations
- The form of an operation is: visibility name (parameter-list) : return-type {prop-string, ...}
where parameters are written as:
direction parameter_name : type [ multiplicity ] = default_value {properties} - Only name is necessary
- visibility ::= { -, +, #, ~}
- direction ::= { in, out, inout}
- An example of this is: + balanceOn (date : Date) : Money {query}
Relationships
Our customer order class diagram has three kinds of relationships.
- association -- a relationship between two classes. There is an association between two classes if an instance of one class must know about the other in order to perform its work. In a diagram, an association is a link connecting two classes.
- aggregation -- an association in which one class (the part-of) belongs to a collection (the whole). An aggregation has a diamond end pointing to the part containing the whole. In our diagram, Order has a collection of OrderDetails so an instance of OrderDetail is a part-of the collection within the class Order.
- generalization -- an inheritance link indicating one class is a superclass of the other. A generalization has a triangle pointing to the superclass. Payment is a superclass of Cash, Check, and Credit.
An association has two ends. An end may have a role name to clarify the nature of the association. For example, an OrderDetail is a line item of each Order.
A navigability arrow on an association shows which direction the association can be traversed or queried. An OrderDetail can be queried about its Item, but not the other way around. The arrow also lets you know who "owns" the association's implementation; in this case, OrderDetail has an Item. Associations with no navigability arrows are bi-directional.
The multiplicity of an association end is the number of possible instances of the class associated with a single instance of the other end. Multiplicities are single numbers or ranges of numbers. In our example, there can be only one Customer for each Order, but a Customer can have any number of Orders.
This table gives the most common multiplicities.
Multiplicities | Meaning |
---|---|
0..1 | zero or one instance. The notation n . . m indicates n to m instances. |
0..* or * | no limit on the number of instances (including none). |
1 | exactly one instance |
1..* | at least one instance |
Every class diagram has classes, associations, and multiplicities. Navigability and roles are optional items placed in a diagram to provide clarity.
Generalization, Association, Aggregation, and Composition
Each of UML relationships represents a different type of connection between classes and has subtleties that aren't fully captured in the UML specification. Be sure that your intended viewers understand what you are conveying with your various relationships.
- Dependency ("uses a" relationship)
The weakest relationship between classes. It means that one class uses, or has knowledge of another class. It is typically a transient relationship. Dependencies are typically read as "...uses a...". E.g., Window uses a WindowClosingEvent. - Association ("has a" relationship)
Associations are stronger then dependencies and typically indicate that one class retains a relationship to another class over an extended period of time. Usually each one can be destroyed without necessarily destroying each other. E.g., Window has a Cursor.- Aggregation ("owns a" relationship)In an aggregation relationship, the part may be independent of the whole but the whole requires the part (containment). E.g., Window owns a Rectangle. An aggregation relationship is indicated in the UML with an unfilled diamond. It can be considered as a shareable aggregation that denotes a weak ownership. That is, the part may be included in several aggregate "owner" classes.
- Composition ("is part of" relationship)A composition relationship, also known as a composite aggregation, is a stronger form of aggregation where the part is created and destroyed with the whole. E.g., Titlebar is part of Window. A composition relationship is indicated in the UML with a filled diamond. The no sharing rule is the key to composition.
- Generalization ("is a" relationship)
A generalization relationship conveys that the target of the relationship is a general, or less specific, version of the source class or interface. E.g., Cat is an Animal.
Notice that with both types of aggregation, the diamond is located on the side of the line pointing to the aggregate class which represents the "whole" in an aggregation (whole-part) relationship.
The following is a handy short-cut guide:
Dependency |
Association
|
Aggregation
| Composition |
Generalization
|
"...uses a..." |
"...has a..."
|
"...owns a..."
| "...is part of..." |
"...is a..."
|
Object Diagrams
Object diagrams show instances instead of classes. They are useful for explaining small pieces with complicated relationships, especially recursive relationships.
This small class diagram shows that a university Department can contain lots of other Departments.
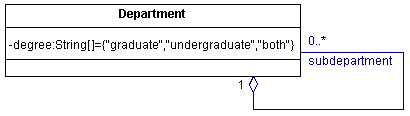
The object diagram below instantiates the class diagram, replacing it by a concrete example.
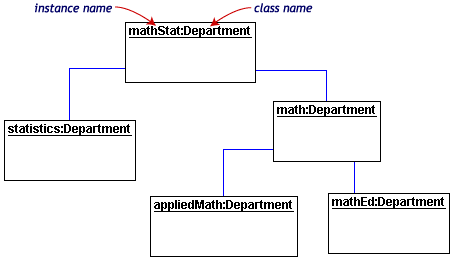
Each rectangle in the object diagram corresponds to a single instance. Instance names are underlined in UML diagrams. Class or instance names may be omitted from object diagrams as long as the diagram meaning is still clear.
Composite Structure Diagrams
A composite structure diagram depicts the internal structure of a classifier in terms of parts.
Part
An element representing a set of instances by composition that are owned by a containing classifier instance or role of a classifier. All such instances are destroyed when the containing classifier is destroyed. Parts may be joined by attached connectors and specify configurations of linked instances to be created within an instance of the containing classifier.
An element representing a set of instances by composition that are owned by a containing classifier instance or role of a classifier. All such instances are destroyed when the containing classifier is destroyed. Parts may be joined by attached connectors and specify configurations of linked instances to be created within an instance of the containing classifier.
Properties in a class diagram and in a structure diagram
A property specifying an instance not owned by composition by the instance of the containing classifier is shown by a graphical nesting of a box symbol with a dashed outline.

Property examples
The namestring of a part obeys the following syntax: <name>‘:’<classifiername> [multiplicity]
The namestring of a part obeys the following syntax: <name>‘:’<classifiername> [multiplicity]

Properties in a structure diagram
Connectors and parts in a structure diagram
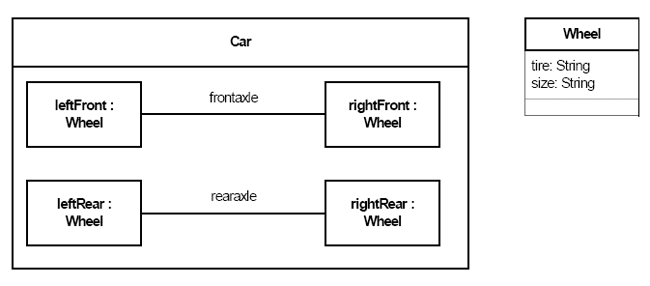
Connectors and parts in a structure diagram using multiplicities

An instance of the Car class
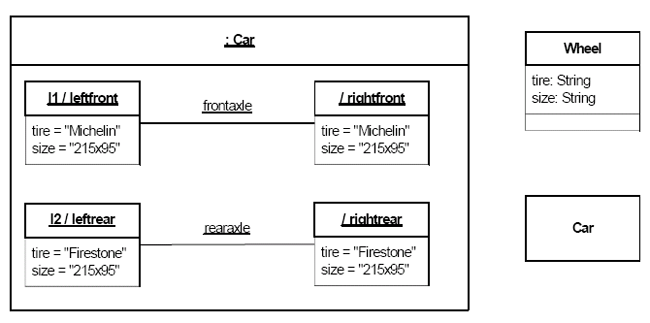
The namestring of a role in an instance specification obeys the following syntax:
{<name> [‘/’ <rolename>] | ‘/’ <rolename>} [‘:’ <classifiername> [‘,’ <classifiername>]*]
The name of the instance specification may be followed by the name of the part that the instance plays. The name of the part may only be present if the instance plays a role.
{<name> [‘/’ <rolename>] | ‘/’ <rolename>} [‘:’ <classifiername> [‘,’ <classifiername>]*]
The name of the instance specification may be followed by the name of the part that the instance plays. The name of the part may only be present if the instance plays a role.
Packages
Examples of packages with members
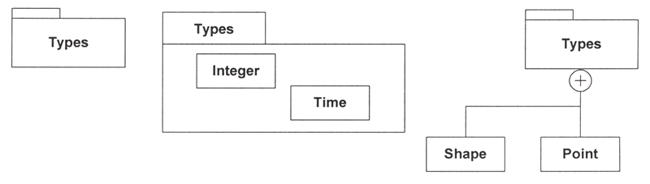
To simplify complex class diagrams, you can group classes into packages. A package is a collection of logically related UML elements. The diagram below is a business model in which the classes are grouped into packages.
Packages and Dependencies

Packages appear as rectangles with small tabs at the top. The package name is on the tab or inside the rectangle. The dotted arrows are dependencies. One package depends on another if changes in the other could possibly force changes in the first.
Packaging Rules
How do you chose which classes to put in which package?
- Common Closure Principle
- Classes in a package should need changing for similar reasons
- Common Reuse Principle
- Classes in a package should be reused together
- Acyclic Dependency Principle
- Avoid cycles in dependencies
- Stable Dependency Principle
- More dependencies coming into package the most stable package’s interface needs to be
- Stable Abstraction Principle
- Stable packages tend to have higher proportion of interfaces and abstract classes
- Stable packages tend to have higher proportion of interfaces and abstract classes
Public and Private Package Imports

Packages - Comments
- Namespace for classes
- The dependency relationships are not transitive
- Indicate global dependency by <<global>>
- Keep application’s dependencies under control
- Reduce the interface of the package by exporting only a small subset of the operations (Facade DP) and make supporting classes private visibility
- A compile-time grouping
- For run-time grouping use component diagrams
Documenting Classes
- Java Language Coding Guidelines
- Code Conventions for the Java Programming Language
- How to Write Doc Comments for the Javadoc Tool
- Eclipse
- Coding Java Style
Window>Preferences>Java>Code StyleFormatting sources: Ctrl+Shift+F or Source>FormatInsert Javadoc comment: Atl+Shift+J - Source code templates
Window>Preferences>Java>Editor>Templates- Using templates
type for example"for"
and press Ctrl+Space to activate Content Assist - Press Tab key to move to the next template parameter
- Shift+Tab to return to the previous parameter
- Using templates
- Spell Checker
Window>General>Editors>Text Editor>Spelling - Javadoc Content Assist
- A Java comment stars with /** and tags with @
- HTML formatting
- Activate Content Assist to get a list of standard HTML tags that can be inserted
- Javadoc View
Window>Show View>Other...>Java>Javadoc - Generate Javadoc
You may select a set of packages, source folders or projects for which you want to generate Javadoc documentation before launching the wizard. The set of elements to generate Javadoc for may also be chosen and modified after the wizard is launched.
Open the Export wizard by doing one of the following:- Selecting Export from the selection's pop-up menu, then Java/Javadoc or
- Selecting File>Export from the menu bar.
- Project>Generate Javadoc...
- Coding Java Style
Tidak ada komentar:
Posting Komentar